本文共 842 字,大约阅读时间需要 2 分钟。
一:安装scala插件:
具体步骤如下:
1:双击IDEA,打开软件
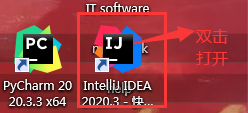
2:进入界面后,点击Plugins
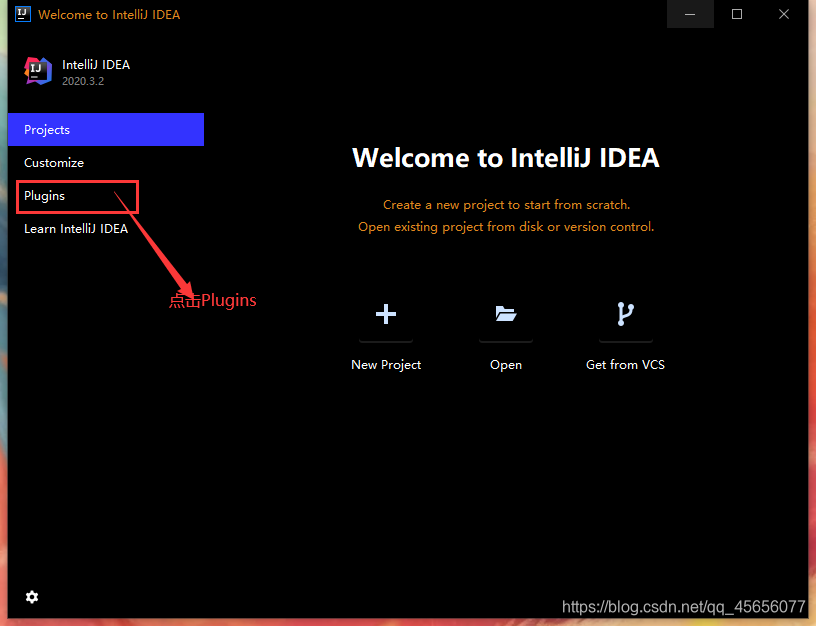
3:在搜索栏输入scala,点击安装
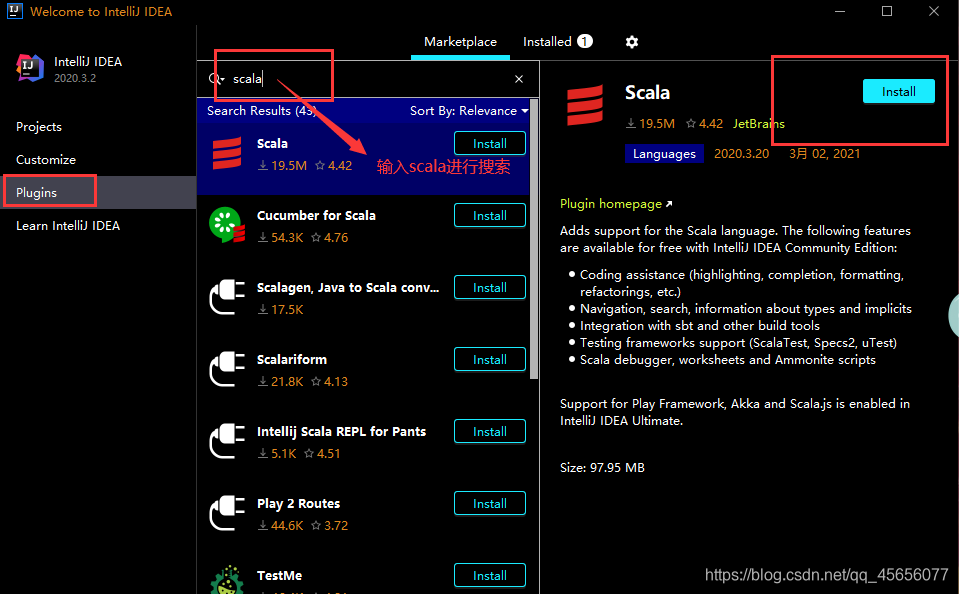
4:正在安装
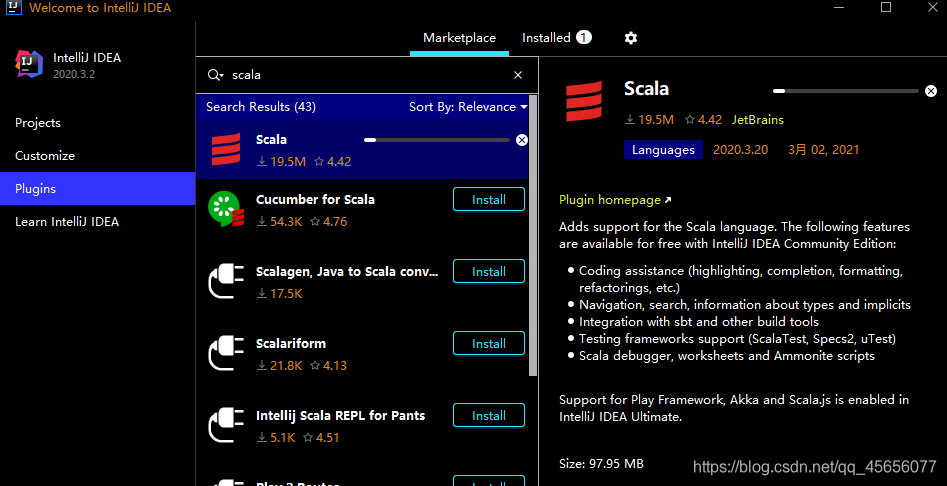
5:也可以到官网进行安装,在网站可查看到scala相对应的版本,然后进行下载即可
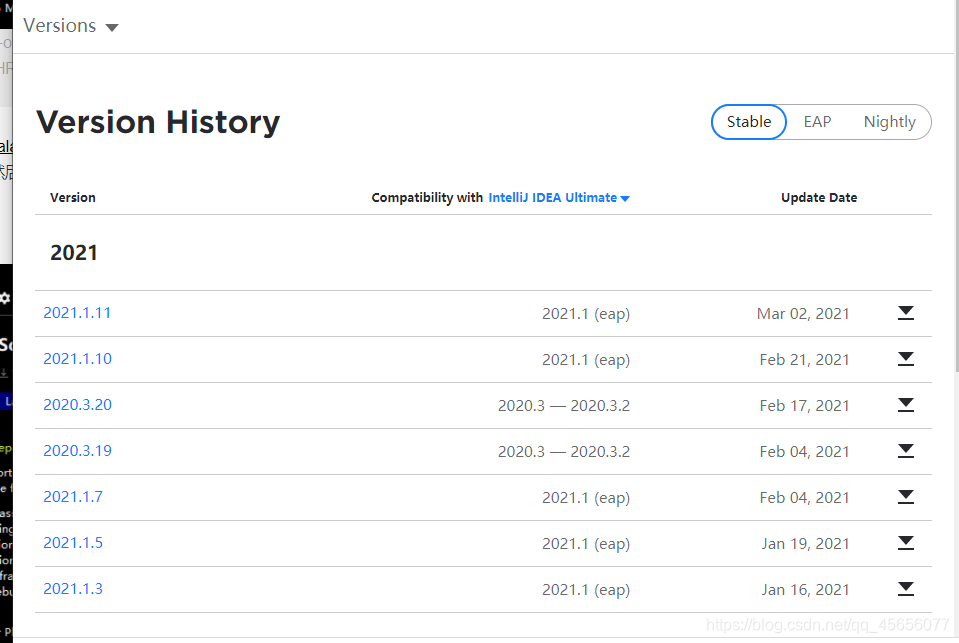
注意:可以在搜索到scala版本后在线安装,但是在线安装很慢,所以建议下载后离线安装
把下载的.zip格式的scala插件放到Intellij的安装的plugins目录下(不用解压),然后加载刚刚放到plugins目录下的.zip文件
点击设置
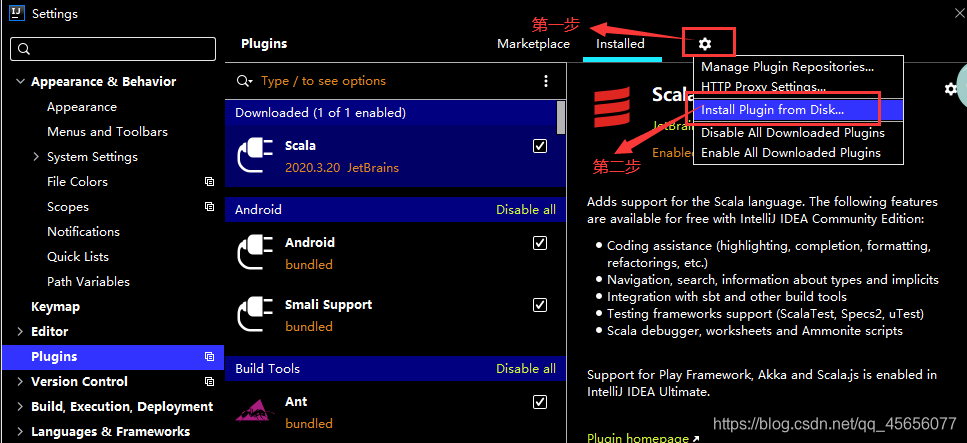
点击后找到你下载的zip文件目录把文件放进去点击OK即可
6:
创建一个scala项目示例:
重点参考scala官方文档:
创建一个scala项目:
1:由于我的IDEA是新安装的还未使用过,使用是下面这模样的,如果已经使用过就点击file—New----project,接下来的步骤就根据第二步执行即可
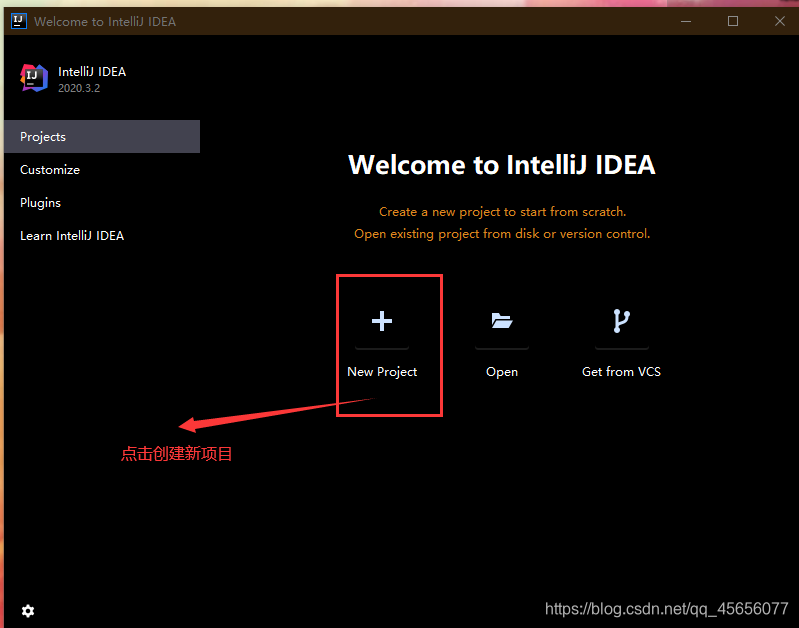
2:在左边导航栏选择scala,然后点击右边的IDEA
3:因为是第一次创建,没有scala SDK,如果默认点击Finish按钮,scala项目建立后将找不到scala class,也就是不能编写scala程序
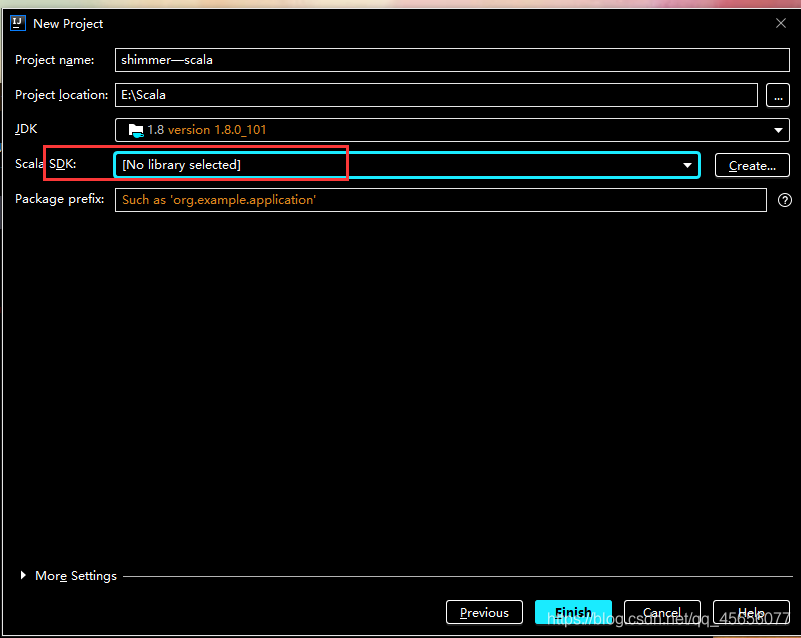
4:因此,需要点击scala SDK右边的create,在弹框中显示了scala SDK版本,可以使用download下载,但是下载很慢,就放弃了
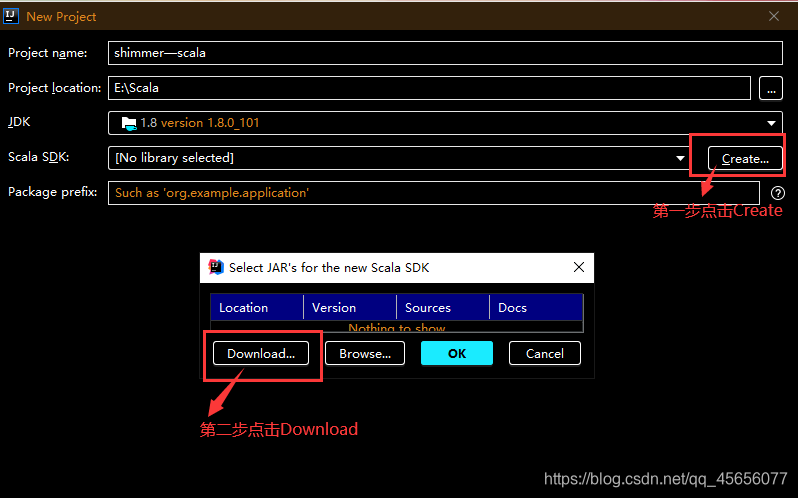
点击Download后的结果,这里有一个好处就是你可以按到你按照的IDEA所使用的scala是哪一个版本的,然后可以根据下面那个步骤去官网下载
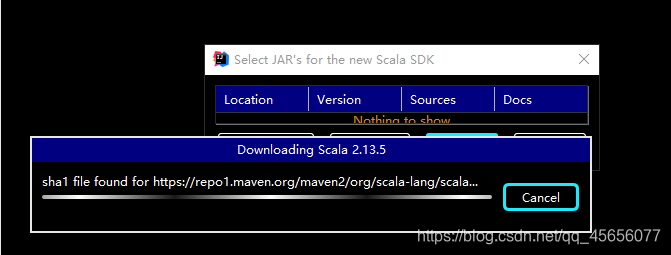
5:
于是采用了第二种方法,前提是安装scala软件,scala的安装包可在网站上下载。点击Browse按钮,在弹出的对话框中加载scala安装后的文件夹。
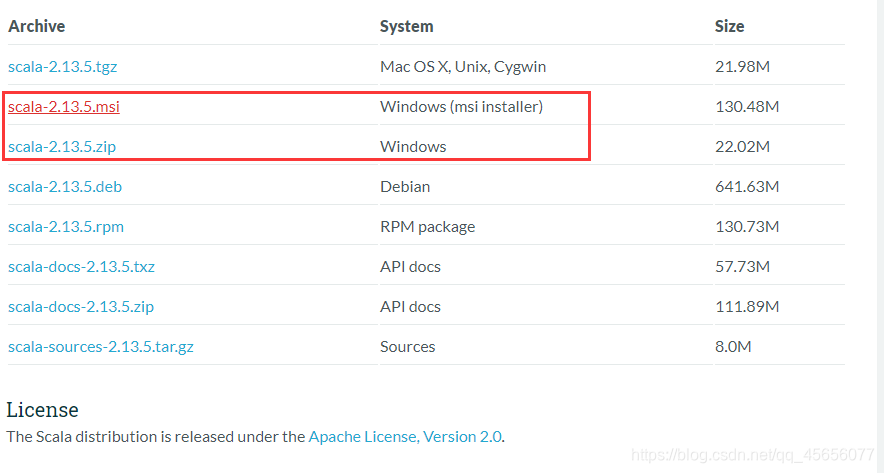
下载完后点击Browse
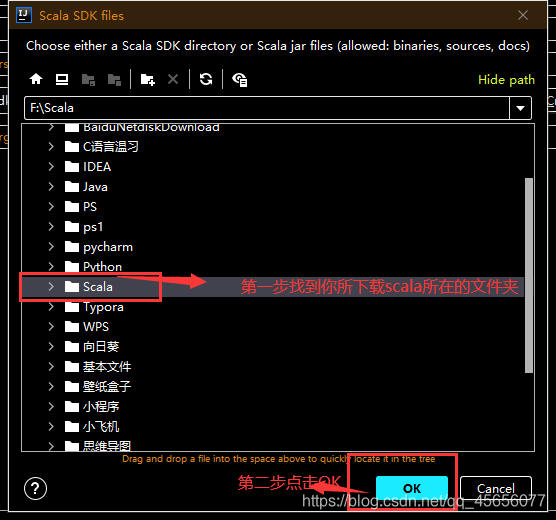
因为我最开始的时候安装了一个scala版本所以我添加进去
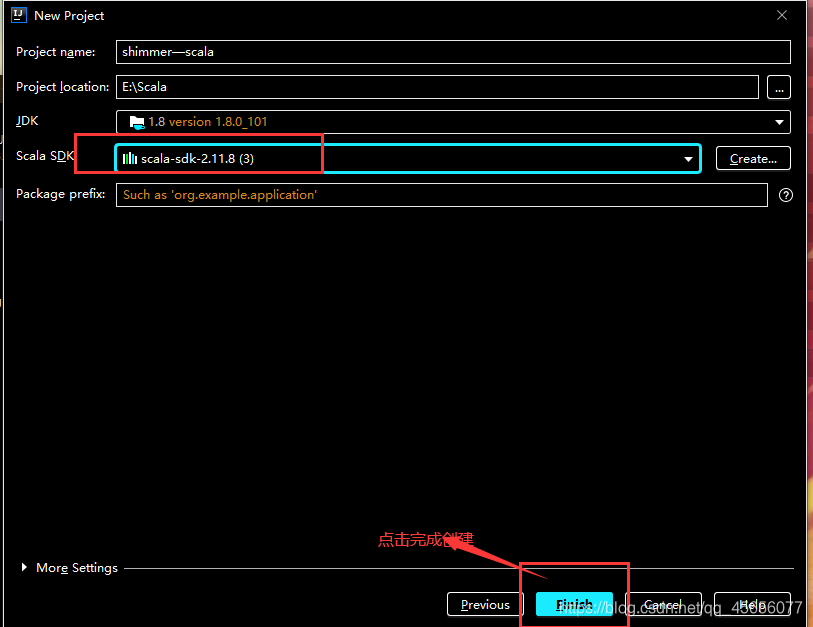
如图,可以创建scala class
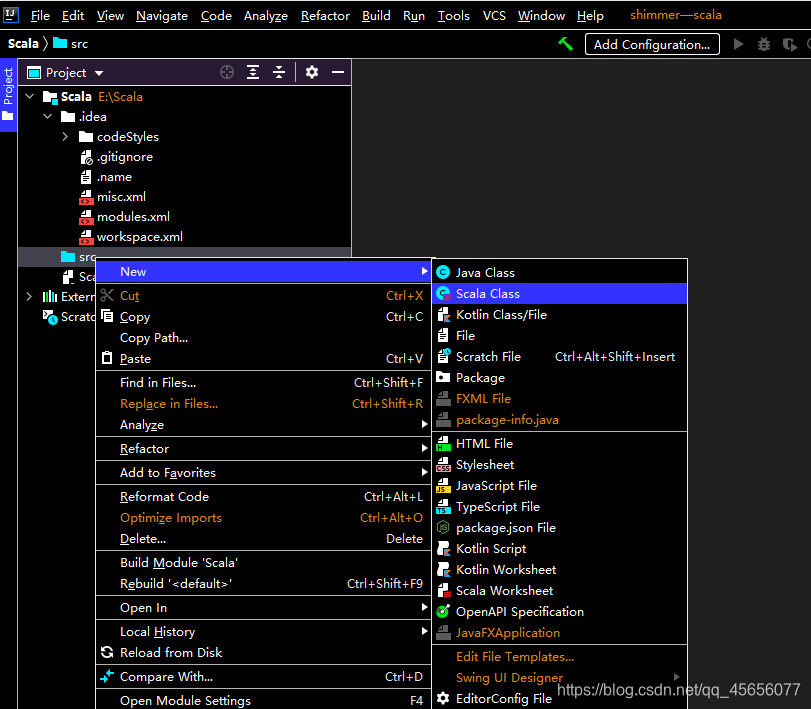
点击OK,完成创建
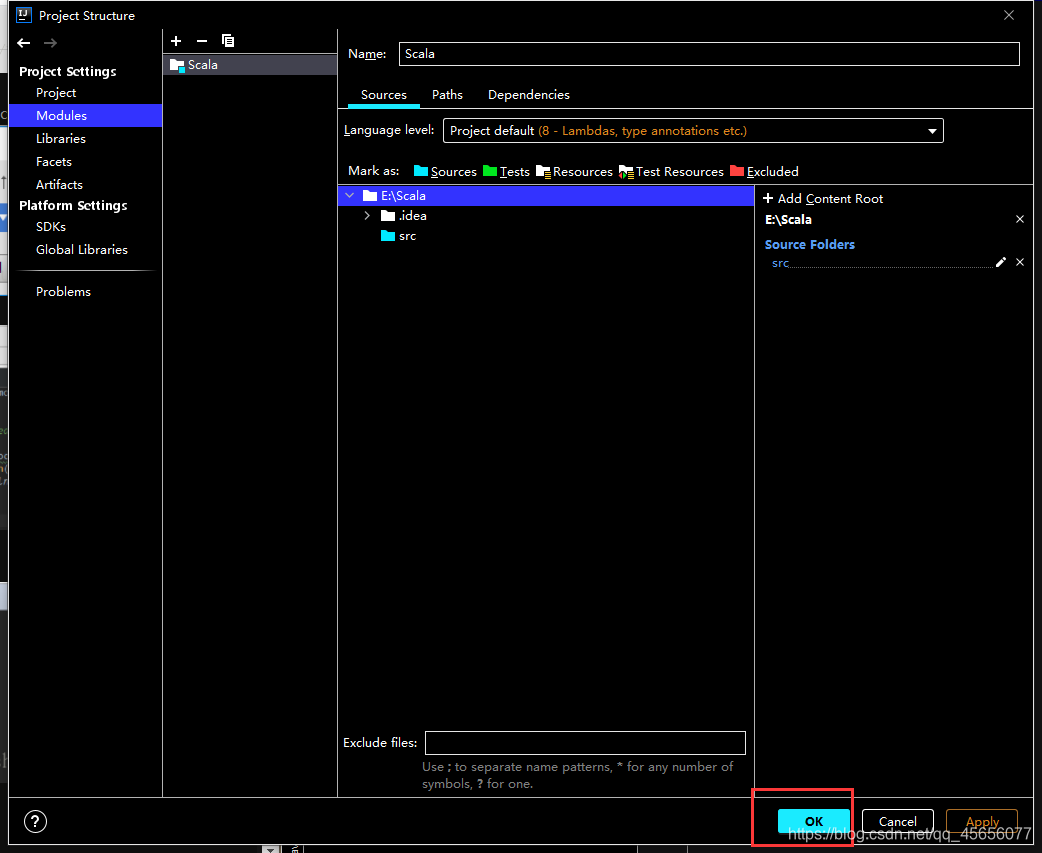
创建一个包:
创建完成,可以敲代码了,欧耶,工欲善其事必先利其器,gogogo》》》》
转载地址:http://vsyg.baihongyu.com/